WordPress is a great CMS for publisher, but there is no built-in function to get and show the primary category for a post.
A post may be under multiple categories, such as “News” and “Recent work”. When you have a list of posts, you might need to show only one category for this post. So, to get this category you will need to place the following function in your functions.php
file.
function get_post_primary_category($post_id, $term='category', $return_all_categories=false){ $return = array(); if (class_exists('WPSEO_Primary_Term')){ // Show Primary category by Yoast if it is enabled & set $wpseo_primary_term = new WPSEO_Primary_Term( $term, $post_id ); $primary_term = get_term($wpseo_primary_term->get_primary_term()); if (!is_wp_error($primary_term)){ $return['primary_category'] = $primary_term; } } if (empty($return['primary_category']) || $return_all_categories){ $categories_list = get_the_terms($post_id, $term); if (empty($return['primary_category']) && !empty($categories_list)){ $return['primary_category'] = $categories_list[0]; //get the first category } if ($return_all_categories){ $return['all_categories'] = array(); if (!empty($categories_list)){ foreach($categories_list as &$category){ $return['all_categories'][] = $category->term_id; } } } } return $return; }
This function assumes the following parameters:
$post_id :
the post ID, for which we want the categories
$term :
By default it is set to ‘category’, but you may set it to any other taxonomy, such as post_tag.
$return_all_categories :
by default it is set to false. However if you want it to return the primary category but also a list of IDs for all of its categories, set it to true. You may use this to check if this post is also under another category. For example if it is also under the category “videos” you can show a video indicator icon for the post.
The function makes use of Yoast SEO plugin, if it is enabled. Yoast SEO plugin adds an extra “primary category” feature if a post has multiple categories.
How to use this function to show in front end:
Simply you can echo
the $primary_category
variable to show it in your WordPress theme.
$post_categories = get_post_primary_category($post->ID, 'category'); $primary_category = $post_categories['primary_category'];
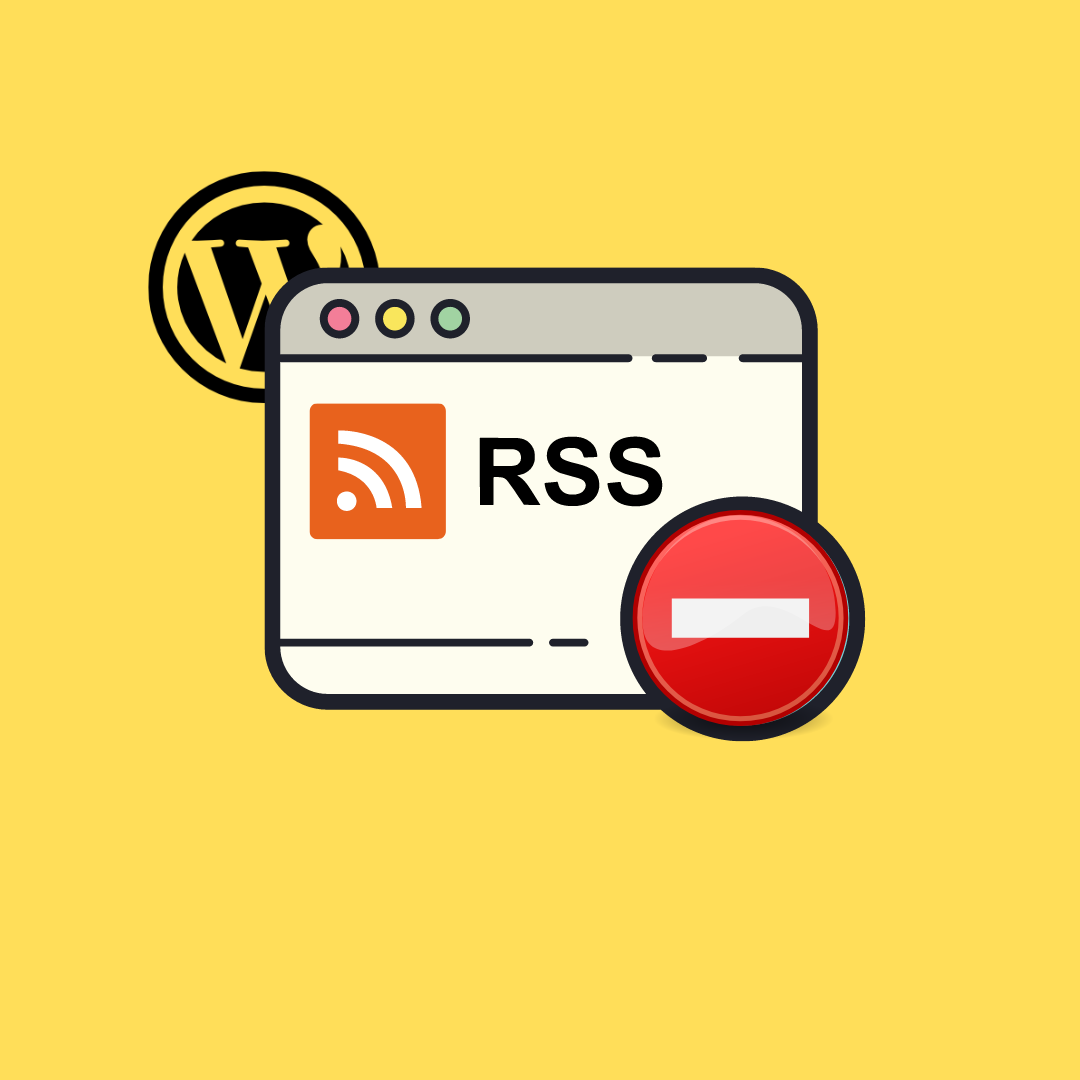